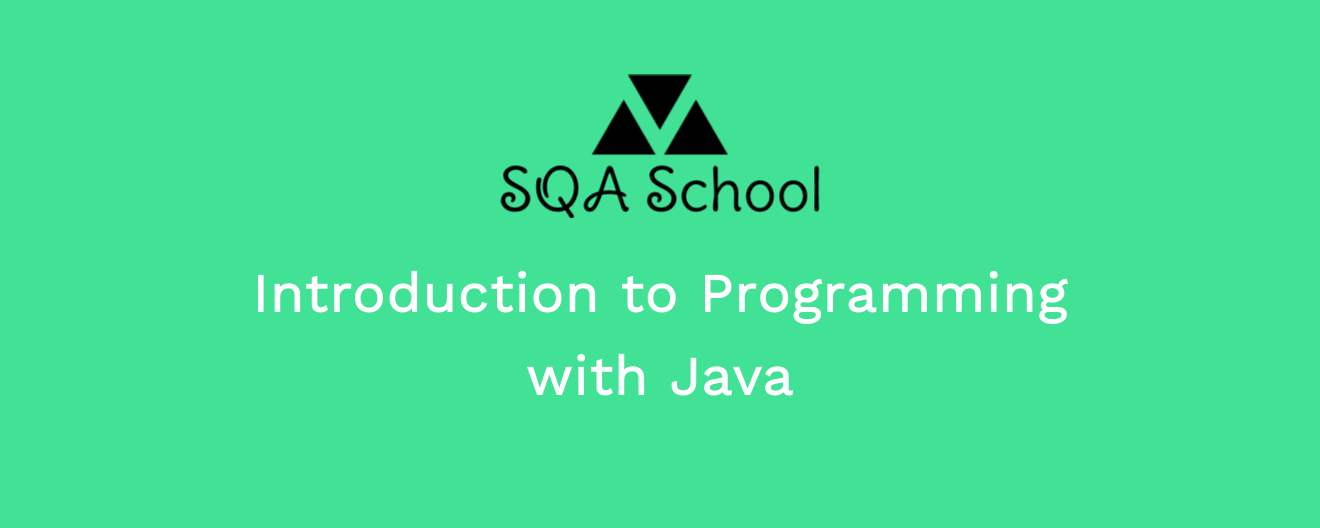
Java Variables and Literals
Java Variables
Variables are named storage locations in memory used to hold data. Each variable in Java must have a unique name and a specific data type, which determines the type of data it can store. Java is case-sensitive, so age
and Age
are recognized as different variables. Java uses the Camel Case convention for variable names, where each word after the first begins with an uppercase letter (e.g., myAge
). Variable names may include letters, digits, underscores, and dollar signs, but they cannot start with a digit or contain spaces.
To create a variable, you declare it by specifying its data type followed by its name, like this:
int age;
Here, age
is a variable of type int
.
You can assign a value to a variable during declaration or later in the code. For instance:
int age = 25;
// or
int age;
age = 25;
Keep in mind that in Java, you need to end a statement with a semicolon.
You can update a variable’s value within the program. For example:
int age = 25;
....
age = 30;
Java Literals
Literals are constant values directly included in your code to represent fixed values of specific data types. For example:
int age = 25;
char aLetter = 'A';
double latitude = 45.0;
Here, 25
, 'A'
, and 45.0
are literals.