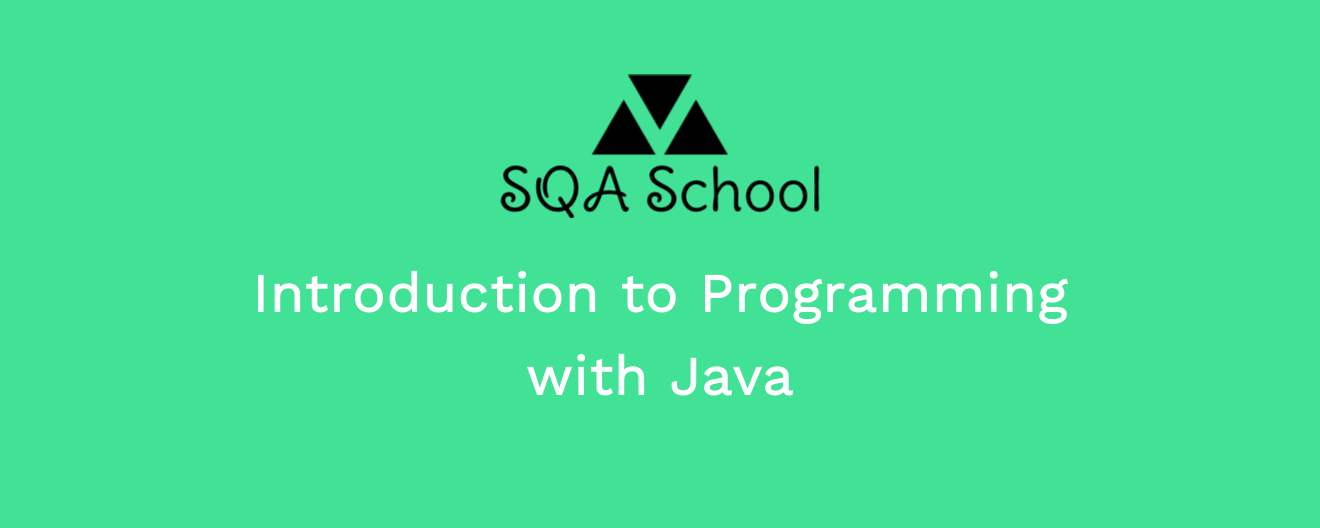
Java Break and Continue
When using loops, there may be times when you want to skip certain actions within the loop or exit the loop altogether. In such cases, the break
and continue
statements come in handy.
break
The break statement is used to exit a loop or switch
statement before it normally completes.
Example
public class Main {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
if (i == 3) {
break; // Exits the loop when i equals 3
}
System.out.println("Iteration " + i + " - Hello, World!");
}
}
}
Output
Iteration 0 - Hello, World!
Iteration 1 - Hello, World!
Iteration 2 - Hello, World!
Take note of the following code:
if (i == 3) {
break; // Exits the loop when i equals 3
}
This means that when i
equals 3
, the loop terminates. As a result, the output only includes values less than 3
.
continue
The continue statement allows you to skip the remaining code in the current iteration of the loop and proceed to the next iteration.
Example
public class Main {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
if (i == 3) {
continue; // Skips when i equals 3
}
System.out.println("Iteration " + i + " - Hello, World!");
}
}
}
Output
Iteration 0 - Hello, World!
Iteration 1 - Hello, World!
Iteration 2 - Hello, World!
Iteration 4 - Hello, World!
Observe this line of code:
if (i == 3) {
continue; // Skips when i equals 3
}
This means that when i
equals 3
, that particular iteration will be bypassed. As a result, the output will not include the value 3
.