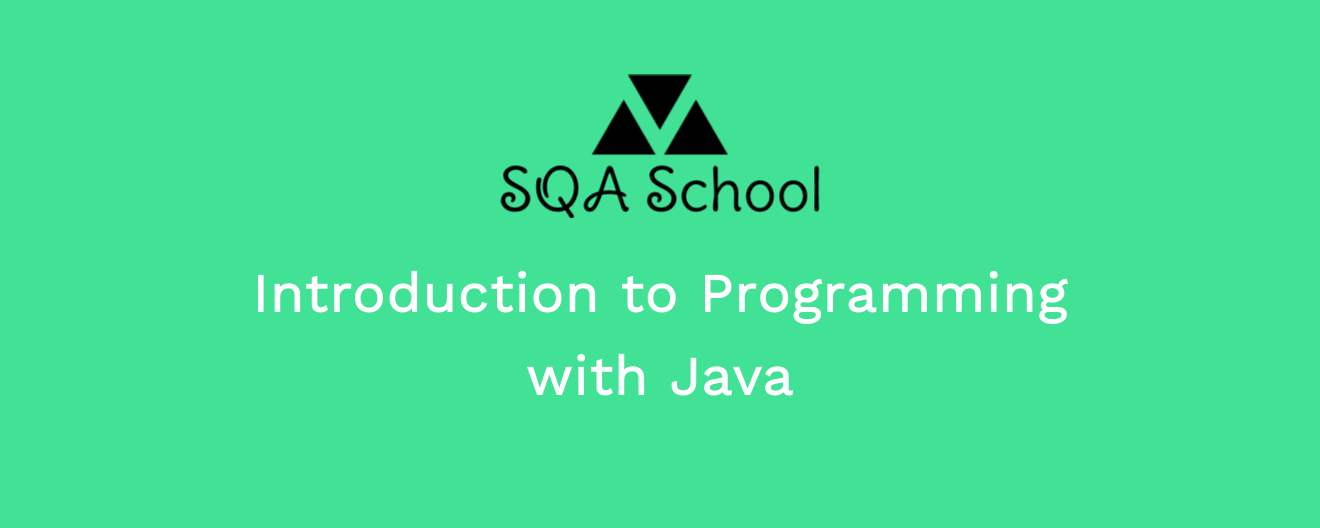
Basic Structure of a Java Program
The first thing to know about Java is that source files have a .java
extension. Java follows Pascal Case for file names, where each word in the name is capitalized (e.g., HelloWorld
). Java is case-sensitive, meaning that HelloWorld
and Helloworld
are considered different class names.
Your Java program must begin with a class definition, where the class name matches the file name.
public class HelloWorld {
...
}
In this example, public class HelloWorld
defines a class named HelloWorld
, saved in a file called HelloWorld.java
. In Java, all code resides within a class.
Next, you need to add the main
method inside the class to run your program’s code. For this tutorial series, it’s important to remember the main
method’s signature: public static void main(String[] args)
. As you learn more about Java’s object-oriented programming concepts, you’ll gain a clearer understanding of each keyword.
public class HelloWorld {
public static void main(String[] args) {
...
}
}
Within the main
method, you’ll write statements that determine your program’s actions. Here, System.out.println()
is used to print Hello, World!
to the console.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Simple enough? We hope it is.
In the following tutorials, we’ll use the template shown below for our code examples.
public class Main {
public static void main(String[] args) {
...
}
}