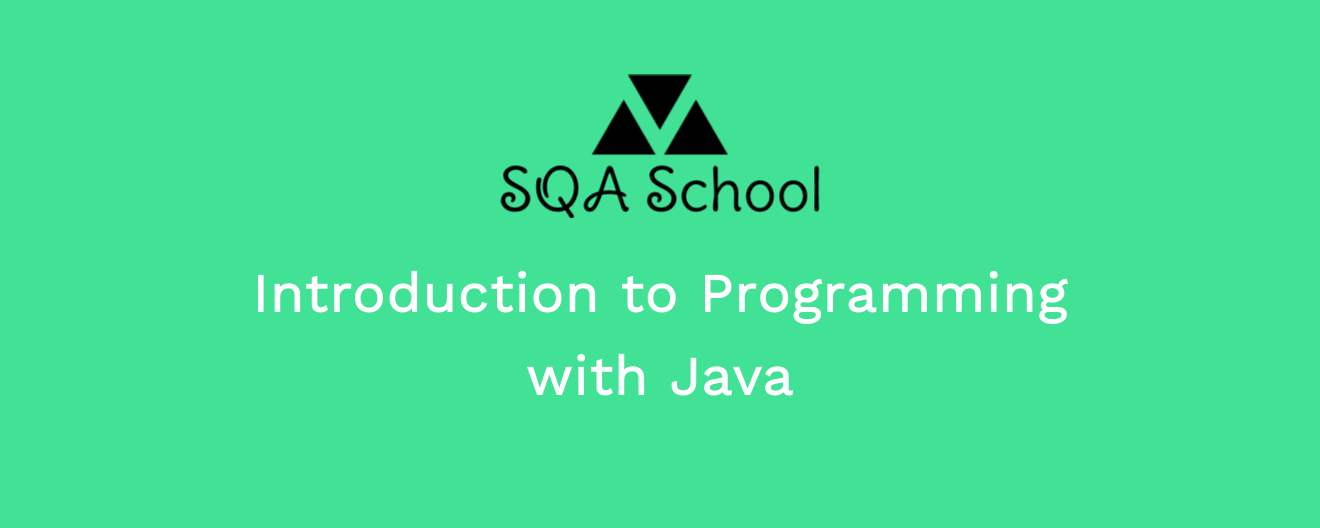
Java Basic Input and Output
Java Input
Java provides several methods to capture user input. In this section, you’ll discover how to collect input from the console using an instance of the Scanner
class. To use the Scanner
class, you must import the java.util.Scanner
package, which should be declared at the beginning of your program, before the class declaration.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
}
}
Next, you need to declare a variable of type Scanner
and instantiate it using new Scanner(System.in)
. In this example, input
is the variable that holds the Scanner
object. Don’t worry if this concept seems unclear at the moment; it will become more understandable as you delve into Java’s object-oriented programming principles.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
}
}
With the input
object ready, you can capture console input by calling its methods. To use a method on the input object, simply add a dot followed by the method name, such as nextInt()
or nextDouble()
.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
byte aByte = input.nextByte(); // captures and stores data of the byte type
short aShort = input.nextShort(); // captures and stores data of the short type
int anInt = input.nextInt(); // captures and stores data of the int type
long aLong = input.nextLong(); // captures and stores data of the long type
float aFloat = input.nextFloat(); // captures and stores data of the float type
double aDouble = input.nextDouble(); // captures and stores data of the double type
boolean aBoolean = input.nextBoolean(); // captures and stores data of the boolean type
String aString = input.next(); // captures and stores data of the String type
System.out.println(aByte);
System.out.println(aShort);
System.out.println(anInt);
System.out.println(aLong);
System.out.println(aFloat);
System.out.println(aDouble);
System.out.println(aBoolean);
System.out.println(aString);
}
}
Input
15
100
12000
9000000000
25.06
89.00987
true
Hello
Output
15
100
12000
9000000000
25.06
89.00987
true
Hello
Java Output
To print output to the console, utilize the System.out.println()
method. Place the text you wish to display inside the parentheses, enclosed in double quotes. If you want to output a variable or a numeric or boolean literal, you do not need quotation marks. For character literals, use single quotes.
public class Main {
public static void main(String[] args) {
int number = 5;
System.out.println(number);
System.out.println(5);
System.out.println('A');
System.out.println("The number is " + number);
}
}
Output
5
5
A
The number is 5
Notice the line System.out.println("The number is " + number);
in the above example. Here, the variable number
is first converted into a string and then concatenated with the text “The number is” using the +
operator.