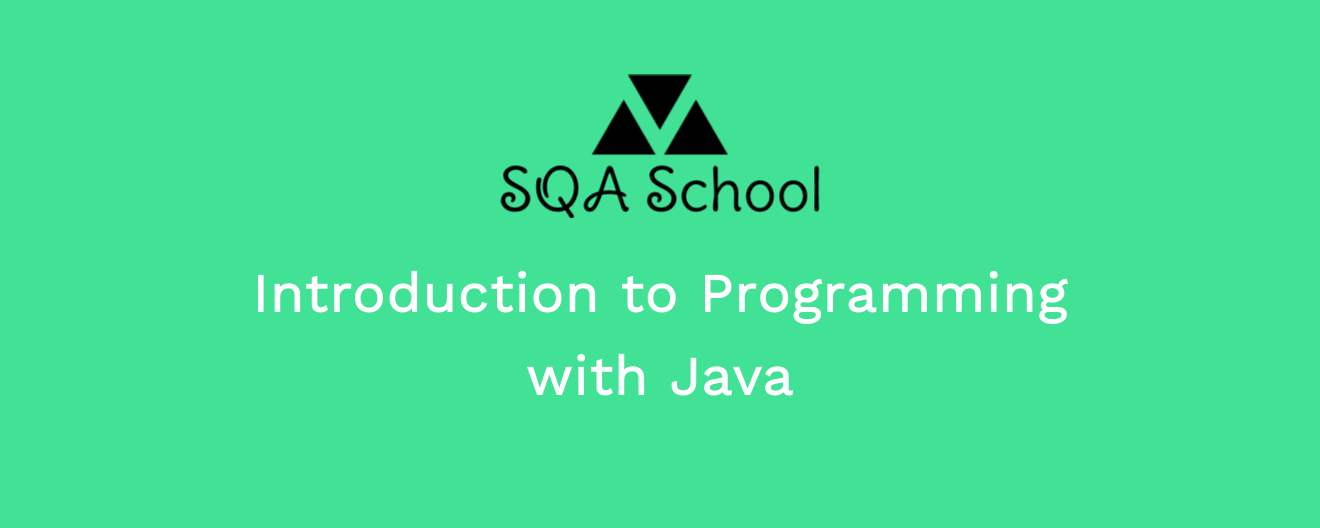
Java Arrays
An array is a structured collection of data elements that share the same type, enabling you to group multiple values under one variable name and access them via an index. For instance, if you want to store the ages of 50 individuals, you can declare an int
array to hold these 50 age values.
To declare an array, you need to specify the data type, followed by square brackets []
, and then give your array a name:
int[] age;
You can then create the array using the new
keyword and specify the number of elements it will contain:
age = new int[50];
Alternatively, you can combine declaration and initialization in a single line:
int[] age = new int[50];
It’s important to note that the size of an array is fixed once it is created. To change the size, you’ll need to create a new array. Additionally, elements in newly created arrays are automatically initialized to default values (e.g., 0
for integers and false
for booleans).
You can assign values to individual elements of an array either separately or at the time of declaration:
int[] age = new int[50];
age[0] = 30;
age[1] = 15;
.....
// or
int[] age = {30, 15, ....};
Array elements can be accessed using zero-based indexing, where the first element is located at index 0
, the second at index 1
, and so forth.
System.out.println(age[0]); // prints 30
To find out how many elements are in an array, you can use the length
property of the array.
System.out.println(age.length); // 50
Here’s a complete example:
public class Main {
public static void main(String[] args) {
// Declaration, creation, and initialization combined
int[] age = {30, 15, 4, 9, 56, 34, 18};
// Retrieving the length of the array
int size = age.length;
// Looping over all the elements of the array
for (int i = 0; i < size; i++) {
// Accessing values from the array
System.out.println("Age " + age[i] + " at index " + i);
}
}
}
Output
Age 30 at index 0
Age 15 at index 1
Age 4 at index 2
Age 9 at index 3
Age 56 at index 4
Age 34 at index 5
Age 18 at index 6