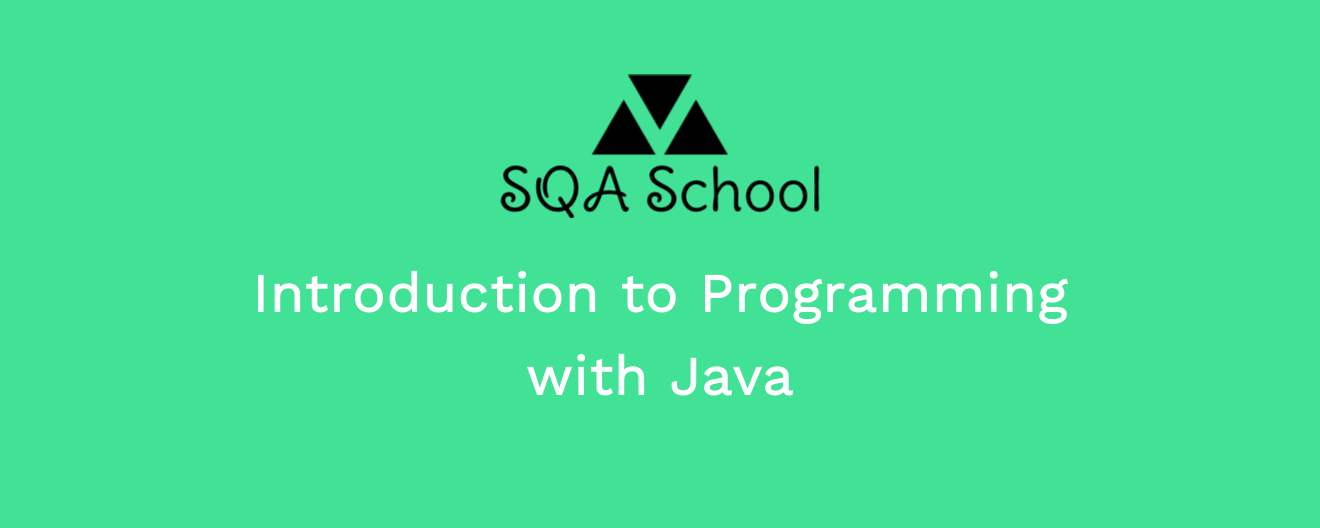
Java Loops
If you want to print "Hello, World!"
to the console 100 times without repeating the System.out.println("Hello, World!");
command, you can utilize loops in Java. A loop repeatedly executes a block of code until a specified termination condition is satisfied. Java provides the following types of loops:
1. for Loop
A for loop is used to execute a block of code a defined number of times. Its syntax is:
for (initialization; condition; update) {
// Code block to be executed
}
- initialization: This expression runs once at the beginning of the loop and is typically used to declare and initialize the loop control variable, for instance:
int i = 0
. - condition: This boolean expression is evaluated before each iteration. If it evaluates to
true
, the code block within the loop is executed; if it’sfalse
, the loop ends. For example:i < 5
. - update: This expression is executed after each iteration and usually updates the loop control variable. For example:
i++
.
Example
public class Main {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
System.out.println("Iteration " + i + " - Hello, World!");
}
}
}
Output
Iteration 0 - Hello, World!
Iteration 1 - Hello, World!
Iteration 2 - Hello, World!
Iteration 3 - Hello, World!
Iteration 4 - Hello, World!
In the above program, the for
loop operates as follows:
Iteration | Explanation |
---|---|
1 | The loop control variable i is initialized to 0, so its value is 0 during the first iteration. Since i is less than 5, the print statement runs. After executing the print statement and before the next iteration, i is incremented by 1, changing its value to 1. |
2 | i is currently 1. Since it is less than 5, the print statement is executed. Once the print statement is executed, i is incremented by 1 before the next iteration, bringing its value to 2. |
3 - 5 | Functions the same way as in the second iteration. |
6 | At this point, i is 5. Because it is not less than 5, the loop ends, and nothing is printed to the console. |
2. while Loop
A while loop executes a block of code repeatedly as long as a specified condition remains true
. Its syntax is:
while (condition) {
// Code block to be executed
}
- condition: This boolean expression is evaluated before each iteration. If it is
true
, the code inside the loop executes; if it isfalse
, the loop stops.
Example
public class Main {
public static void main(String[] args) {
int i = 0;
while (i < 5) {
System.out.println("Iteration " + i + " - Hello, World!");
i++;
}
}
}
Output
Iteration 0 - Hello, World!
Iteration 1 - Hello, World!
Iteration 2 - Hello, World!
Iteration 3 - Hello, World!
Iteration 4 - Hello, World!
In this program, the while
loop works as follows:
Iteration | Explanation |
---|---|
1 | The loop control variable i is set to 0 before the while loop begins, so in the first iteration its value is 0. Since i is less than 5, the print statement is executed first, and then i is incremented to 1. |
2 | i is currently 1. Since it is less than 5, the print statement is executed, and then i is incremented by 1, bringing its value to 2. |
3 - 5 | Functions the same way as in the second iteration. |
6 | At this point, i is 5. Because it is not less than 5, the loop ends, and nothing is printed to the console. |
3. do-while Loop
A do-while loop guarantees that a block of code runs at least once and continues to execute as long as a specified condition is true. This type of loop ensures the code executes at least once, even if the initial condition is false. Its syntax is:
do {
// Code block to be executed
} while (condition);
- condition: After executing the code block, the
condition
is checked. If it istrue
, the loop repeats; if it isfalse
, the loop stops.
Example
public class Main {
public static void main(String[] args) {
int i = 0;
do {
System.out.println("Iteration " + i + " - Hello, World!");
i++;
} while (i < 0);
}
}
Output
Iteration 0 - Hello, World!
In this program, the do-while
loop operates as follows:
Iteration | Explanation |
---|---|
1 | The loop control variable i is initialized to 0 before the do-while loop starts, so it begins with a value of 0 in the first iteration. The print statement runs first, followed by incrementing i to 1. At this stage, the condition i < 0 is checked. Since it is false, the loop terminates. |