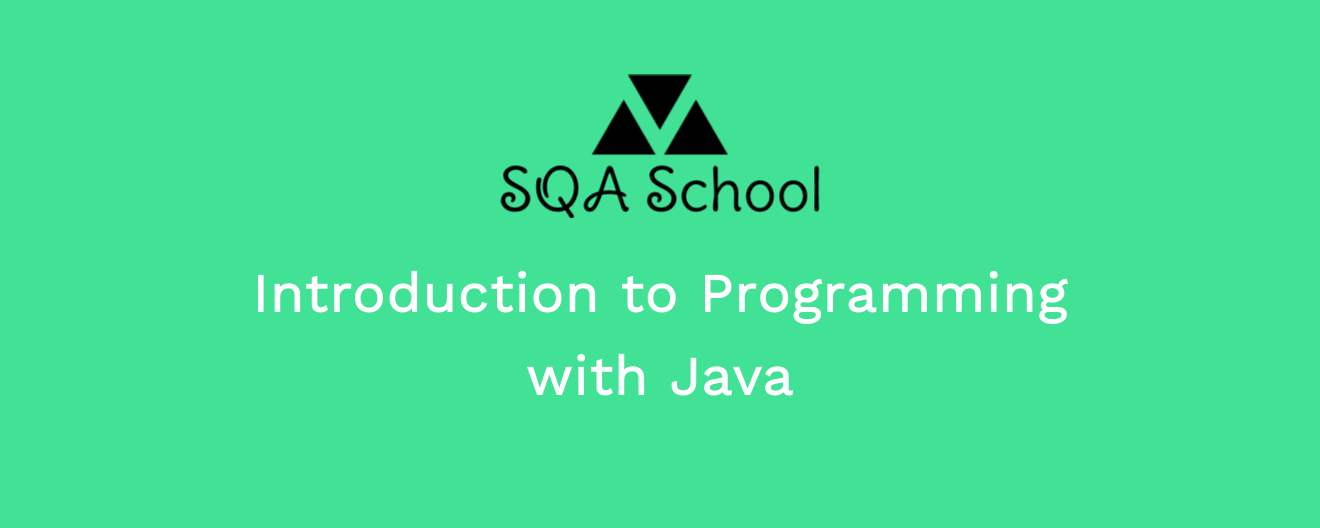
Java Conditional Statements
Conditional statements allow you to run a specific block of code out of multiple options based on certain conditions. They help manage the flow of execution within a Java program. Below are the main types of conditional statements in Java:
1. if
The syntax of an if statement is:
if (condition) {
// Code to be executed if condition evaluates to true
}
Here, condition
is a boolean expression. If the condition
evaluates to true
, the code inside the if
block will be executed. If the condition is false
, the code within the if
block will be skipped.
Example
public class Main {
public static void main(String[] args) {
int age = 25;
if (age > 20) {
System.out.println("You are older than 20.");
}
}
}
Output
You are older than 20.
2. if-else
The syntax of an if-else statement is:
if (condition) {
// Code to be executed if condition evaluates to true
} else {
// Code to be executed if condition evaluates to false
}
In this case, condition
is again a boolean expression. If the condition
is true
, the code in the if
block executes; if it’s false
, the code in the else
block runs.
Example
public class Main {
public static void main(String[] args) {
int age = 18;
if (age > 20) {
System.out.println("You are older than 20.");
} else {
System.out.println("You are younger than 20.");
}
}
}
Output
You are younger than 20.
3. if-else if-else
The if-else if-else statement allows you to check multiple conditions, each of which is a boolean expression. The code corresponding to the first true
condition will execute, and if no conditions are met, the final else
block will run. The syntax is:
if (condition1) {
// Code to be executed if condition1 evaluates to true
} else if (condition2) {
// Code to be executed if condition2 evaluates to true
} else {
// Code to be executed if none of the conditions are true
}
Example
public class Main {
public static void main(String[] args) {
int age = 18;
if (age > 20) {
System.out.println("You are older than 20.");
} else if (age > 15) {
System.out.println("You are older than 15.");
} else {
System.out.println("You're younger.");
}
}
}
Output
You are older than 15.
4. switch
The switch statement offers a clearer and more compact alternative to using if-else if-else
statements when handling multiple possible values for a single variable. Its syntax is:
switch (expression) {
case value1:
// Code to be executed if expression matches value1
break;
case value2:
// Code to be executed if expression matches value2
break;
default:
// Code to be executed if no case matches
}
Here, the expression
is evaluated once and compared against the values of each case
. If the expression
matches value1
, the code under case value1:
is executed. Similarly, if the expression matches value2
, the code under case value2:
is run. If there is no match, the code under default:
will be executed.
Example
public class Main {
public static void main(String[] args) {
int age = 25;
switch (age) {
case 30:
System.out.println("You are 30 years old.");
break;
case 25:
System.out.println("You are 25 years old.");
break;
default:
System.out.println("You are neither 30 nor 20 years old.");
}
}
}
Output
You are 25 years old.
5. Ternary Operator
The ternary operator provides a compact way to write an if-else
statement. The syntax is:
variable = (condition) ? value1 : value2;
Here, variable
is assigned value1
if the condition is true
, and value2
otherwise.
Example
public class Main {
public static void main(String[] args) {
int age = 25;
boolean isOver20 = (age > 20) ? true : false;
System.out.println(isOver20);
}
}
Output
true