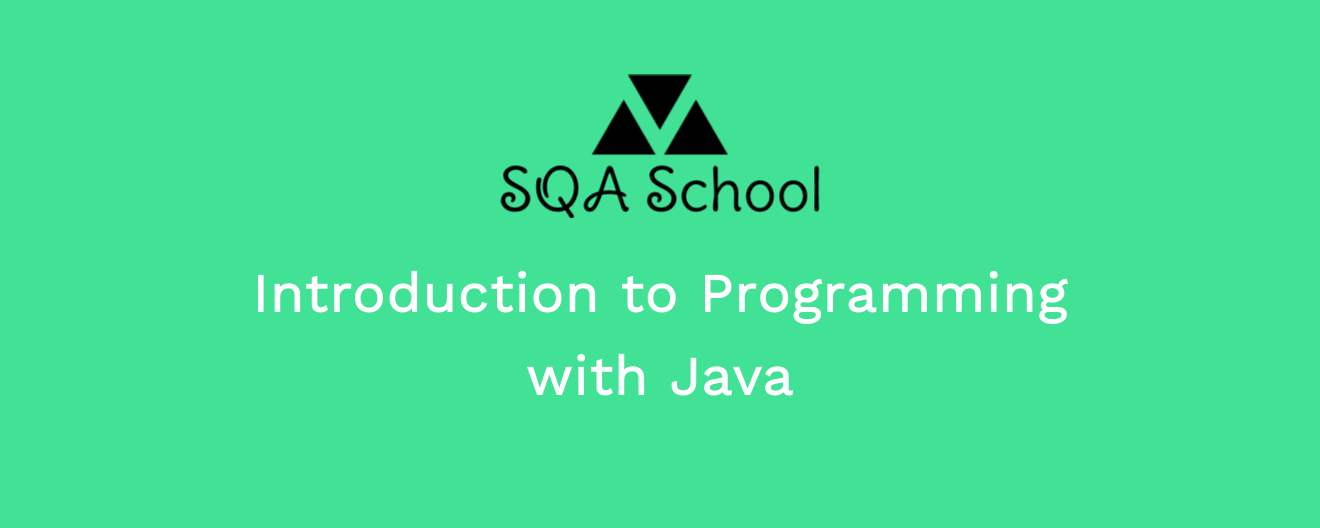
Java Primitive Data Types
In the previous tutorials, we covered variables, literals, and keywords. You learned that to declare a variable, you must specify both its data type and name, and the name cannot be a reserved Java keyword. This tutorial will introduce you to the different data types available for variables in Java.
Data types define the type of data a variable can hold. In Java, data types are categorized into primitive and reference types. In this series, we’ll focus on primitive data types.
Java has eight primitive data types:
1. byte
The byte type is an 8-bit signed integer that can hold values from -128
to 127
, with 0
as its default value.
public class Main {
public static void main(String[] args) {
byte number = 127;
System.out.println(number);
}
}
Output
127
2. short
The short type is a 16-bit signed integer, storing values between -32,768
and 32,767
, with 0
as its default.
public class Main {
public static void main(String[] args) {
short number = 32767;
System.out.println(number);
}
}
Output
32767
3. int
The int type is a 32-bit signed integer, with a range from -2,147,483,648
to 2,147,483,647
and a default value of 0
.
public class Main {
public static void main(String[] args) {
int number = 2147483647;
System.out.println(number);
}
}
Output
2147483647
4. long
The long type is a 64-bit signed integer, storing values from –9,223,372,036,854,775,808
to 9,223,372,036,854,775,807
, with a default of 0
.
public class Main {
public static void main(String[] args) {
long number = 9223372036854775807L;
System.out.println(number);
}
}
Output
9223372036854775807
Notice the L
at the end, which denotes that this is a long
literal.
5. float
The float type is a 32-bit single-precision floating-point number for decimal values, with a default of 0.0f
.
public class Main {
public static void main(String[] args) {
float number = 50.25f;
System.out.println(number);
}
}
Output
50.25
The f
suffix indicates that 50.25
is a float
literal; without it, Java would interpret the number as a double
.
6. double
The double type is a 64-bit double-precision floating-point number for decimal values, with 0.0
as the default value.
public class Main {
public static void main(String[] args) {
double number = 50.25;
System.out.println(number);
}
}
Output
50.25
7. char
The char type is a 16-bit Unicode character, with values ranging from \u0000
to \uffff
and a default of \u0000
. char
literals should be enclosed in single quotes. Assigning an integer to a char
will display the character corresponding to that ASCII value.
public class Main {
public static void main(String[] args) {
char aDigit = '\u0031'; // represents the Unicode character '1'
char aVowel = 'A'; // represents the character 'A'
char aLetter = 72; // represents the character 'H'
System.out.println(aDigit);
System.out.println(aVowel);
System.out.println(aLetter);
}
}
Output
1
A
H
8. boolean
The boolean type holds one of two values: true or false, with false
as the default. It’s commonly used in conditional statements.
public class Main {
public static void main(String[] args) {
boolean isTrue = true;
System.out.println(isTrue);
}
}
Output
true