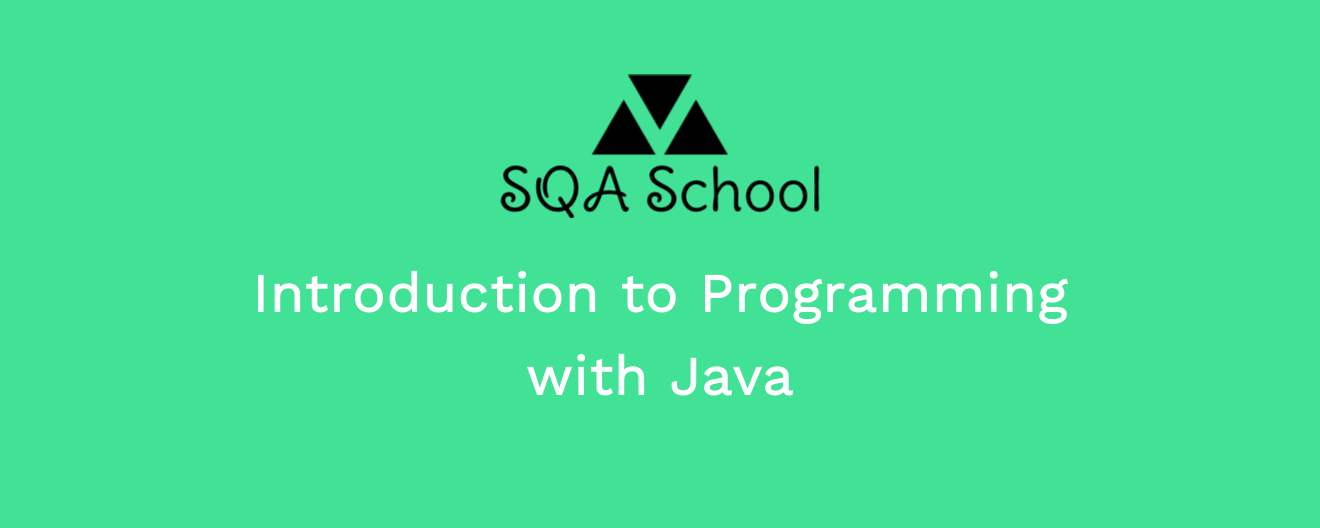
Java for-each Loop
Now that you’ve learned about arrays in Java, let’s explore the for-each loop, also known as the enhanced for loop. This loop is ideal for iterating over elements in arrays and collections (a framework providing various interfaces and classes for data structures and algorithms). In this tutorial, we’ll focus on how to use it with arrays.
The syntax for the for-each loop is:
for (dataType variableName : dataStructure) {
...
}
In this syntax:
- dataType: Specifies the type of elements in the array or collection.
- variableName: A variable assigned to each item from the array or collection during each iteration.
- dataStructure: The array or collection being traversed.
Example
public class Main {
public static void main(String[] args) {
int[] age = {30, 15, 4, 9, 56, 34, 18};
for (int eachAge : age) {
System.out.println("Age: " + eachAge);
}
}
}
Output
Age: 30
Age: 15
Age: 4
Age: 9
Age: 56
Age: 34
Age: 18