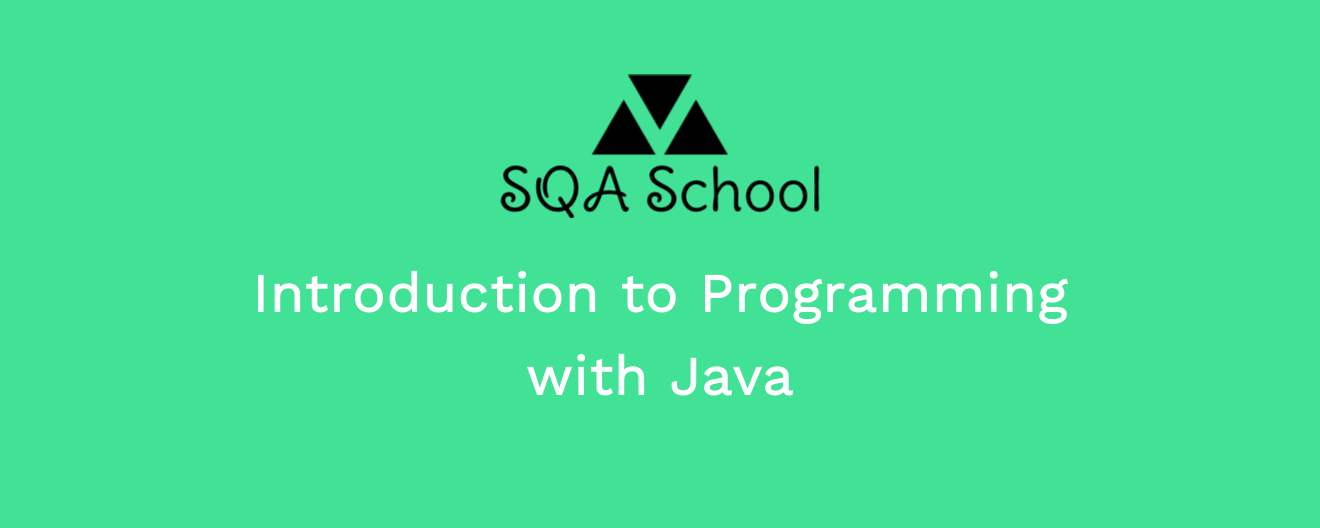
Java Strings
In Java, a string is a sequence of characters. For instance, Hello, World!
is a string made up of the characters 'H'
, 'e'
, 'l'
, 'l'
, 'o'
, ','
, ' '
, 'W'
, 'o'
, 'r'
, 'l'
, 'd'
, and '!'
. To create a string, you enclose text within double quotes:
String greeting = "Hello, World!";
Java also allows creating strings using the new
keyword, which you’ll understand better as you learn about Java’s object-oriented concepts.
Strings in Java are immutable, meaning they cannot be modified after creation. If you perform an operation that appears to change a string, a new string is actually created. Unlike primitive types (like int
or char
), strings are objects of the String
class, so every string variable is an instance of this class.
Basic String Methods
To use a method on a String
object, just type a dot after the string, followed by the method name. Here are some commonly used methods:
length():
Returns the number of characters in the string.
String text = "Hello";
int length = text.length(); // 5
equals():
Checks if two strings have the same value.
String text1 = "Hello";
String text2 = "hello";
boolean isEqual = text1.equals(text2); // false
charAt(index):
Retrieves the character at a specific position (index starts at 0).
String text = "Hello";
char c = text.charAt(1); // 'e'
toUpperCase()
/toLowerCase()
: Converts all characters to uppercase or lowercase.
String text = "Hello";
String upper = text.toUpperCase(); // "HELLO"
concat()
or+
: Combines two strings.
String greeting = "Hello, " + "World!"; // "Hello, World!"
Escape Characters in Strings
Escape characters in strings allow you to include special characters, such as double quotes, within the text.
For example, suppose you want to include double quotes in a string:
String example = "This is an "example".";
This code will cause an error because the compiler will only interpret "This is an "
as the string. To fix this, use the escape character \
to include the quotes within the string:
String example = "This is an \"example\".";
Other common escape characters in Java include:
\n
- Newline\'
- Single quote\\
- Backslash\t
- Tab\r
- Carriage return