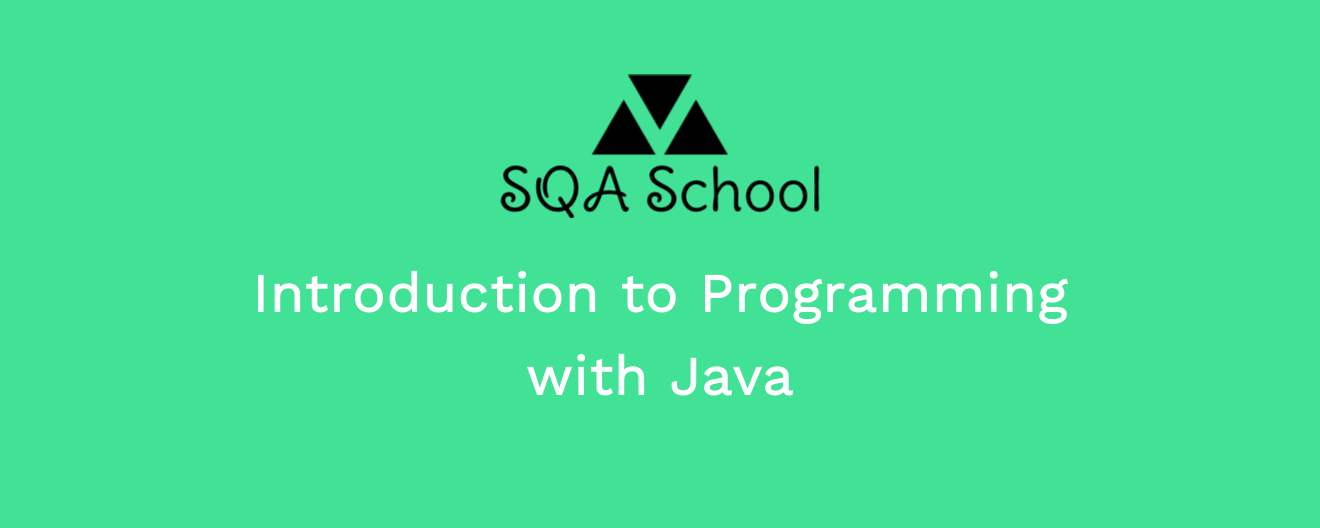
Java Operators
Now that you’ve learned about literals and how to declare and initialize variables, let’s dive into using them alongside operators.
Operators are symbols that let you perform operations on values or expressions. In Java, these operations work on variables, literals, or even entire expressions. Operators are classified into the following categories:
1. Arithmetic Operators
Arithmetic operators perform basic math operations. Here’s a list of arithmetic operators:
Operator | Meaning | Example |
---|---|---|
+ | Adds two operands. | a + b |
- | Subtracts the second operand from the first. | a - b |
* | Multiplies two operands. | a * b |
/ | Divides the first operand by the second. | a / b |
% | Returns the remainder of the division of the first operand by the second. | a % b |
Example
public class Main {
public static void main(String[] args) {
int a = 3;
int b = 2;
System.out.println(a + b); // 3 + 2 = 5
System.out.println(a - b); // 3 - 2 = 1
System.out.println(a * b); // 3 * 2 = 6
System.out.println(a / b); // 3 / 2 = 1
System.out.println(a % b); // 3 % 2 = 1
}
}
Output
5
1
6
1
1
When dividing integers, the result will also be an integer. However, if one operand is a floating-point number, the result will be a floating-point number.
Example
public class Main {
public static void main(String[] args) {
System.out.println(3 / 2);
System.out.println(3.0 / 2);
System.out.println(3 / 2.0);
System.out.println(3.0 / 2.0);
}
}
Output
1
1.5
1.5
1.5
2. Assignment Operators
Assignment operators are used to set values for variables. The fundamental assignment operator is the equals sign, but Java offers various assignment operators that apply an operation to a variable before assigning the outcome to it. The following table presents these operators:
Operator | Meaning | Example |
---|---|---|
= | Assigns the value on the right to the variable on the left. | a = b |
+= | Equivalent to a = a + b. | a += b |
-= | Equivalent to a = a - b. | a -= b |
*= | Equivalent to a = a * b. | a *= b |
/= | Equivalent to a = a / b. | a /= b |
%= | Equivalent to a = a % b. | a %= b |
Example
public class Main {
public static void main(String[] args) {
int a;
int b;
a = 3;
b = 2;
a = b;
System.out.println(a); // 2
a = 3;
b = 2;
a += b; // a = a + b, i.e, a = 3 + 2
System.out.println(a); // 5
a = 3;
b = 2;
a -= b; // a = a - b, i.e, a = 3 - 2
System.out.println(a); // 1
a = 3;
b = 2;
a *= b; // a = a * b, i.e, a = 3 * 2
System.out.println(a); // 6
a = 3;
b = 2;
a /= b; // a = a / b, i.e, a = 3 / 2
System.out.println(a); // 1
a = 3;
b = 2;
a %= b; // a = a % b, i.e, a = 3 % 2
System.out.println(a); // 1
}
}
Output
2
5
1
6
1
1
3. Logical Operators
Logical operators are used to determine if an expression is true
or false
. Below are the logical operators in Java:
Operator | Meaning | Example |
---|---|---|
&& | Evaluates to true if both operands are true; otherwise, it evaluates to false. | a && b |
|| | Evaluates to true if at least one of the operands is true; if both are false, it evaluates to false. | a || b |
! | Inverts the value of the operand. If the operand is true, it becomes false, and if it’s false, it becomes true. | !a |
Example
public class Main {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println(a && b); // true && false = false
System.out.println(a || b); // true || false = true
System.out.println(!a); // !true = false
}
}
Output
false
true
false
4. Relational Operators
Relational operators are used to compare two operands and determine the relationship between them. These operators return a boolean value based on whether the comparison is true or false. The following table lists the relational operators:
Operator | Meaning | Example |
---|---|---|
== | Checks if two operands are equal. | a == b |
!= | Checks if two operands are not equal. | a != b |
> | Checks if the left operand is greater than the right operand. | a > b |
< | Checks if the left operand is less than the right operand. | a < b |
>= | Checks if the left operand is greater than or equal to the right operand. | a >= b |
<= | Checks if the left operand is less than or equal to the right operand. | a <= b |
Example
public class Main {
public static void main(String[] args) {
int a = 3;
int b = 2;
System.out.println(a == b); // 3 == 2 = false
System.out.println(a != b); // 3 != 2 = true
System.out.println(a > b); // 3 > 2 = true
System.out.println(a < b); // 3 < 2 = false
System.out.println(a >= b); // 3 >= 2 = true
System.out.println(a <= b); // 3 <= 2 = false
}
}
Output
false
true
true
false
true
false
5. Unary Operators
Unary operators operate on a single operand to perform various operations such as incrementing or decrementing a value, inverting a boolean value, or changing the sign of a number. The following table lists the unary operators:
Operator | Meaning | Example |
---|---|---|
+ | Indicates a positive value, usually redundant as numbers are positive by default. | +a |
- | Negates the value of the operand, turning a positive number into a negative one, and vice versa. | -a |
++ | Increases the value of the operand by 1. There are two types: 1. Pre-Increment: Increments the value before using it in an expression. 2. Post-Increment: Uses the value in an expression before incrementing it. |
++a, a++ |
−− | Deccreases the value of the operand by 1. There are two types: 1. Pre-Decrement: Decrements the value before using it in an expression. 2. Post-Decrement: Uses the value in an expression before decrementing it. |
−−a, a−− |
! | Inverts the value of a boolean expression. If the operand is true, it becomes false, and if it’s false, it becomes true. | !a |
~ | Inverts all the bits of the operand, effectively changing each 0 to 1 and each 1 to 0. | ~a |
Example
public class Main {
public static void main(String[] args) {
byte a = 3;
boolean b = true;
System.out.println(+a); // +3 = 3
System.out.println(-a); // -3
System.out.println(++a); // a = a + 1 then System.out.println(a), i.e, a = 4 then prints 4
System.out.println(a++); // System.out.println(a) then a = a + 1, i.e, prints 4 then a = 5
System.out.println(--a); // a = a - 1 then System.out.println(a), i.e, a = 4 then prints 4
System.out.println(a--); // System.out.println(a) then a = a - 1, i.e, prints 4 then a = 3
System.out.println(!b); // !true = false
System.out.println(~a); // ~00000011 = 11111100 = -4
}
}
Output
3
-3
4
4
4
4
false
-4
6. Bitwise Operators
Bitwise operators work at the binary level, manipulating the bits directly within the operands. The following table lists the bitwise operators:
Operator | Meaning | Example |
---|---|---|
& | Performs a logical AND operation on each pair of corresponding bits of two operands. | a & b |
| | Performs a logical OR operation on each pair of corresponding bits of two operands. | a | b |
^ | Performs a logical XOR operation on each pair of corresponding bits of two operands. | a ^ b |
~ | Inverts all the bits of the operand. | ~a |
<< | Shifts all bits of the operand to the left by the specified number of positions, filling the rightmost bits with 0s. | a << b |
>> | Shifts all bits of the operand to the right by the specified number of positions, filling the leftmost bits with the sign bit (preserving the sign of the number). | a >> b |
>>> | Shifts all bits of the operand to the right by the specified number of positions, filling the leftmost bits with 0s regardless of the sign of the original number. | a >>> b |
Example
public class Main {
public static void main(String[] args) {
byte a = 6; // 00000110 (in Binary)
byte b = 10; // 00001010 (in Binary)
/*
* 00000110
* 00001010
* -------- (AND)
* 00000010 = 2 (in Decimal)
*/
System.out.println(a & b);
/*
* 00000110
* 00001010
* -------- (OR)
* 00001110 = 14 (in Decimal)
*/
System.out.println(a | b);
/*
* 00000110
* 00001010
* -------- (XOR)
* 00001100 = 12 (in Decimal)
*/
System.out.println(a ^ b);
/*
* 00000110
* -------- (NOT)
* 11111001 = -7 (in Decimal)
*/
System.out.println(~a);
/*
* 00000110
* -------- (Left Shift by 1)
* 00001100 = 12 (in Decimal)
*/
System.out.println(a << 1);
/*
* 00000110
* -------- (Right Shift by 1)
* 00000011 = 3 (in Decimal)
*/
System.out.println(a >> 1);
/*
* 00000110
* -------- (Unsigned Right Shift by 1)
* 00000011 = 3 (in Decimal)
*/
System.out.println(a >>> 1);
}
}
Output
2
14
12
-7
12
3
3
7. instanceof Operator
The instanceof operator checks if an object is an instance of a specified class or a subclass. It returns true
or false
.
Example
public class Main {
public static void main(String[] args) {
String helloWorld = "Hello, World!";
System.out.println(helloWorld instanceof String);
}
}
Output
true
8. Ternary Operator
Ternary operator (?:
) is a shorthand way of writing a simple if-else
statement. It is the only operator in Java that takes three operands, hence the name “ternary.” The ternary operator is used to evaluate a boolean expression and return one of two values depending on whether the expression is true
or false
.
Example
public class Main {
public static void main(String[] args) {
int a = 3;
int b = 2;
System.out.println(a > b ? a : b); // (if 3 > 2 then 3, else 2) = 3
}
}
Output
3