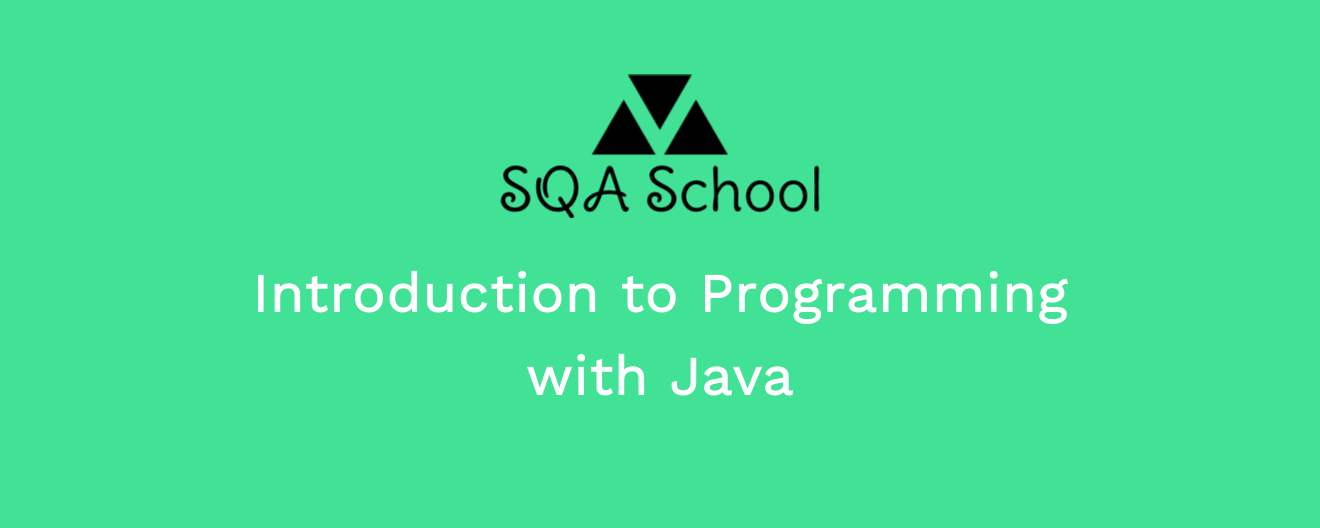
Java Comments
In Java, you might want to add notes or explanations within your code to clarify specific sections for future reference. Java allows this through comments, which the compiler ignores. There are three types of comments in Java:
1. Single-Line Comment
Single-line comments are ideal for brief notes on a single line and start with //
.
public class Main {
public static void main(String[] args) {
// prints "Hello, World!" to the console
System.out.println("Hello, World!");
}
}
Output
Hello, World!
2. Multi-Line Comment
Multi-line comments allow for longer descriptions across multiple lines. They begin with /*
and end with */
.
public class Main {
public static void main(String[] args) {
/* prints "Hello, World!"
to the console */
System.out.println("Hello, World!");
}
}
Output
Hello, World!
3. Documentation Comment
Documentation comments are used to generate external documentation for your code. They start with /**
and end with */
, and are typically placed before classes, methods, or fields. The Javadoc tool uses these comments to produce HTML documentation.
public class Main {
/**
* @param args String array
* prints "Hello, World!" to the console
*/
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Output
Hello, World!