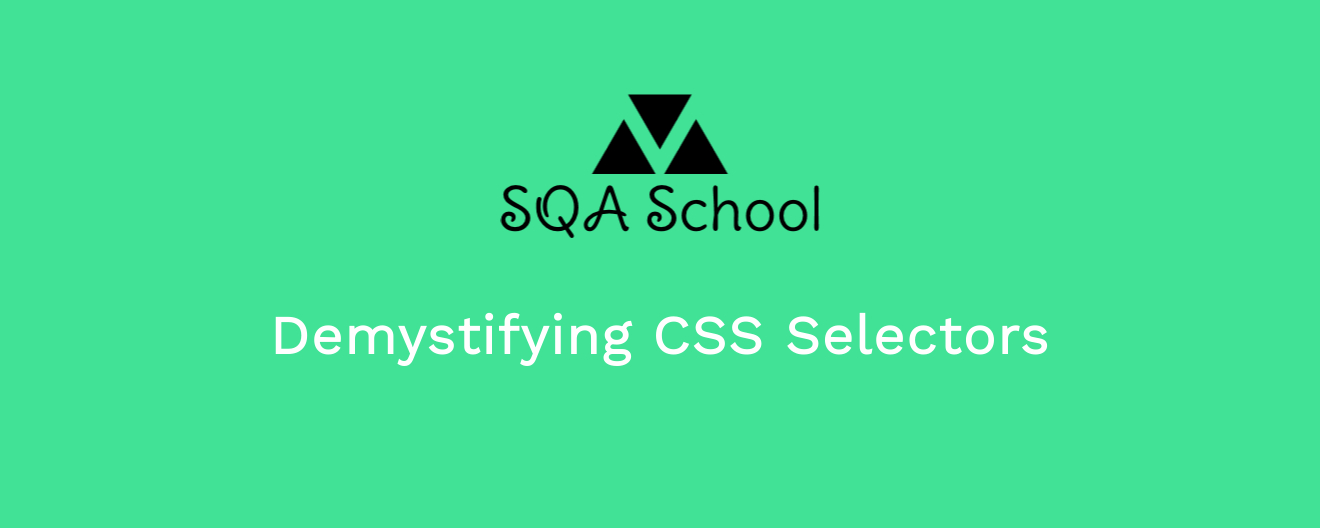
CSS Pseudo-Class Selectors
CSS pseudo-class selectors allow you to select web elements based on specific states or conditions. Like CSS attribute selectors, pseudo-class selectors can be combined with tag names, classes, or IDs for more precise targeting. The following pseudo-class selectors are particularly useful in test automation:
1. :focus
The :focus selector identifies web elements that are currently in focus, such as input fields or buttons. This state is activated when the element is clicked, tapped, or accessed via the Tab
key. For example:
<!DOCTYPE html>
<html>
<body>
<form>
<label>Full Name: <input name="fullName" type="text" /></label>
</form>
</body>
</html>

In this HTML document, the input:focus
selector targets the text field when it is in focus.

2. :checked
The :checked selector targets checkboxes, radio buttons, or option elements that are currently selected. An element is considered checked when it has the “checked” attribute. For example:
<!DOCTYPE html>
<html>
<body>
<form>
<p>Which fruit do you prefer?</p>
<label><input name="favoriteFruit" type="radio" value="orange" checked/>Orange</label>
<label><input name="favoriteFruit" type="radio" value="apple" />Apple</label>
</form>
</body>
</html>
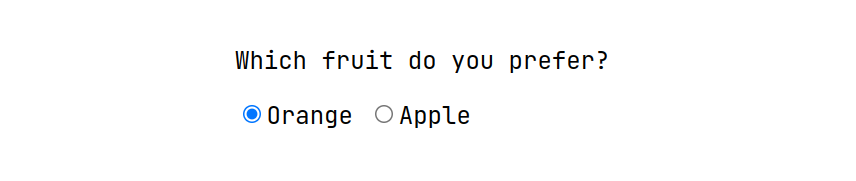
Here, the input:checked
selector will identify the radio button for “Orange.”
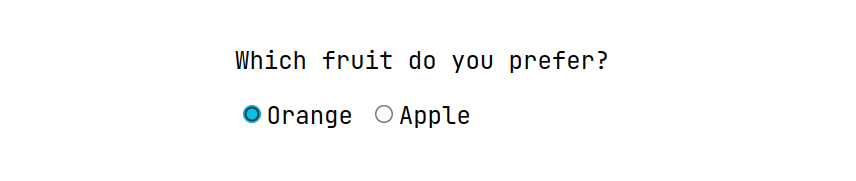
3. :disabled
The :disabled selector identifies elements that are currently disabled, meaning they have the “disabled” attribute. For example:
<!DOCTYPE html>
<html>
<body>
<button type="button" disabled>This is a button (Disabled)</button>
</body>
</html>

In this document, the button:disabled
selector will target the disabled button.

4. :enabled
The :enabled selector locates elements that are enabled—those that can be interacted with, receive focus, and don’t have the “disabled” attribute. For example:
<!DOCTYPE html>
<html>
<body>
<button type="button">This is a button (Enabled)</button>
</body>
</html>

Here, the button:enabled
selector will select the enabled button.

5. :first-child
The :first-child selector targets the first element within a group of sibling elements. For example:
<!DOCTYPE html>
<html>
<body>
<ul>
<li>Red</li>
<li>Green</li>
<li>Blue</li>
</ul>
</body>
</html>
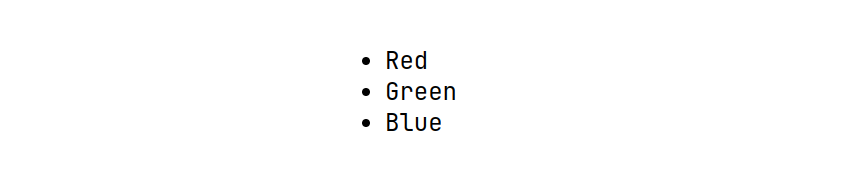
In this example, the li:first-child
selector targets the “Red” list item.
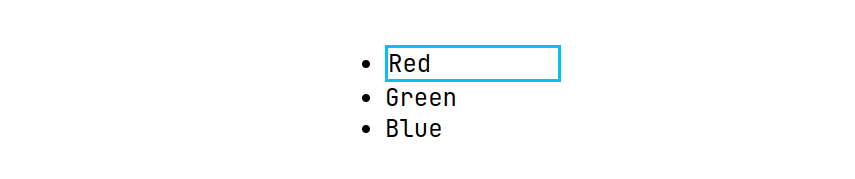
6. :last-child
The :last-child selector targets the last element within a group of sibling elements. For example:
<!DOCTYPE html>
<html>
<body>
<ul>
<li>Red</li>
<li>Green</li>
<li>Blue</li>
</ul>
</body>
</html>
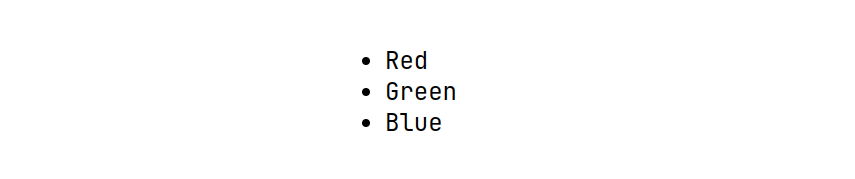
Here, the li:last-child
selector targets the “Blue” list item.
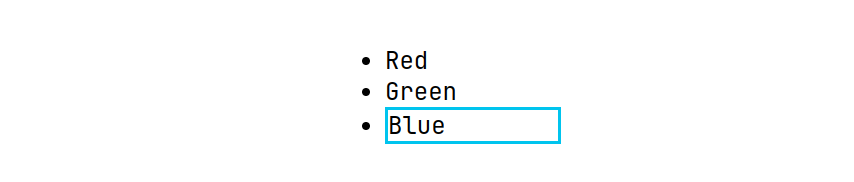
7. :nth-child(n)
The :nth-child(n) selector targets elements based on their position among siblings. For example:
<!DOCTYPE html>
<html>
<body>
<ul>
<li>Red</li>
<li>Green</li>
<li>Blue</li>
</ul>
</body>
</html>
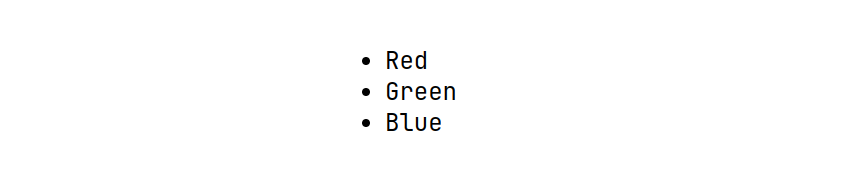
Here, the li:nth-child(2)
selector targets the “Green” list item.
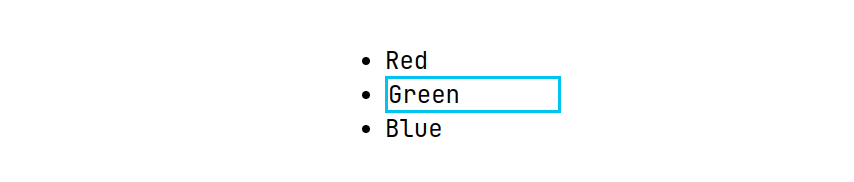
8. :not(selector)
The :not(selector)
selector targets elements that do not match the given selector. For example:
<!DOCTYPE html>
<html>
<body>
<p class="first">First paragraph</p>
<p>Second paragraph</p>
</body>
</html>

In this HTML document, the p:not(.first)
selector identifies the second paragraph.
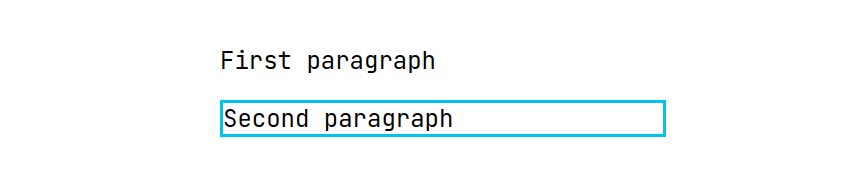